Polymorphism
Moderator: Coders of Rage
- hurstshifter
- ES Beta Backer
- Posts: 713
- Joined: Mon Jun 08, 2009 8:33 pm
- Favorite Gaming Platforms: SNES
- Programming Language of Choice: C/++
- Location: Boston, MA
- Contact:
Polymorphism
Had my first taste of polymorphism in C++ the other day in my summer session class. I've got to admit that I'm thrilled at its possibilities and am really glad (if only for learning inheritance and polymophism) that I took this class.
That is all. Discuss amongst yourselves. I'll give you a topic: the chickpea is neither a chick, nor a pea.
That is all. Discuss amongst yourselves. I'll give you a topic: the chickpea is neither a chick, nor a pea.
"Time is an illusion. Lunchtime, doubly so."
http://www.thenerdnight.com
http://www.thenerdnight.com
- dandymcgee
- ES Beta Backer
- Posts: 4709
- Joined: Tue Apr 29, 2008 3:24 pm
- Current Project: https://github.com/dbechrd/RicoTech
- Favorite Gaming Platforms: NES, Sega Genesis, PS2, PC
- Programming Language of Choice: C
- Location: San Francisco
- Contact:
Re: Polymorphism
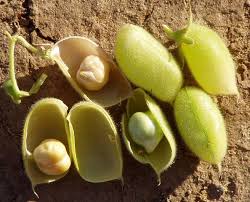
Polymorphism and inheritance are both cool concepts, but I still haven't figured out when it's appropriate to use them.
I've found my habits often evolve much more around composition, and (rarely) aggregation.
This is probably a bad thing, and in the future I hope to learn to successfully apply these methods in tandem.

Falco Girgis wrote:It is imperative that I can broadcast my narcissistic commit strings to the Twitter! Tweet Tweet, bitches!
Re: Polymorphism
Inheritance and polymorphism are two of the main ingredients in object oriented programming. If you're not using them; you might as well just use plain old C.dandymcgee wrote:
Polymorphism and inheritance are both cool concepts, but I still haven't figured out when it's appropriate to use them.
I've found my habits often evolve much more around composition, and (rarely) aggregation.
This is probably a bad thing, and in the future I hope to learn to successfully apply these methods in tandem.
(Excuse the C# code here, my C++ is a bit rusty)
Imagine you had a class, for example class Car.
Code: Select all
public class Car
{
public string Name;
public virtual void Buy()
{
FindOnEbay();
}
}
Code: Select all
public class Ford : Car
{
public string Model;
public int HorsePower;
public override void Buy()
{
BuyFromDealership(Name, Model);
}
}
Re: Polymorphism
this is exactly why there is a influx of sub par programmers. they think that using every feature of language,
or implementing every know design pattern or design methodology makes them great programmers, or have
the attitude that if someone doesn't they are sub par programmers or should stick to a "other" language...
or implementing every know design pattern or design methodology makes them great programmers, or have
the attitude that if someone doesn't they are sub par programmers or should stick to a "other" language...
Some person, "I have a black belt in karate"
Dad, "Yea well I have a fan belt in street fighting"
Dad, "Yea well I have a fan belt in street fighting"
- hurstshifter
- ES Beta Backer
- Posts: 713
- Joined: Mon Jun 08, 2009 8:33 pm
- Favorite Gaming Platforms: SNES
- Programming Language of Choice: C/++
- Location: Boston, MA
- Contact:
Re: Polymorphism
Not sure who this was being directed at but I don't think anyone in this thread has expressed the need to use every feature of the language nor has anyone claimed that they are better programmers for knowing polymorphism. As for me, I literally just learned this concept and it was interesting for me as I am very new to OO design. I think that people looking down on other programmers who over-utilize OO design techniques is just as counter-productive as the OO-abusers themselves. While it is definitely important to know when and where to use each feature of the language, it's also important to know how to use these features. I think we can agree on that.avansc wrote:this is exactly why there is a influx of sub par programmers. they think that using every feature of language,
or implementing every know design pattern or design methodology makes them great programmers, or have
the attitude that if someone doesn't they are sub par programmers or should stick to a "other" language...
EDIT: "If you're not using them; you might as well just use plain old C." - Sorry, I noticed this comment afterwards. This I do not agree with.
"Time is an illusion. Lunchtime, doubly so."
http://www.thenerdnight.com
http://www.thenerdnight.com
Re: Polymorphism
You seem to have quite a high-horse attitude. By no means did I state: 'You're not using OOP to it's fullest. Go use C'. I stated a fact, and an opinion. Then gave an example of how to use something he isn't quite familiar with. (Assuming so at least)avansc wrote:this is exactly why there is a influx of sub par programmers. they think that using every feature of language,
or implementing every know design pattern or design methodology makes them great programmers, or have
the attitude that if someone doesn't they are sub par programmers or should stick to a "other" language...
Using every feature of the language, or every known design pattern/methodology, usually means your code is over-complicated (especially when you consider C++).
Inheritance and polymorphism is what makes OOP so useful.
Also, I'm not sure how you have the knowledge, or ability, to call someone a sub-par programmer. Especially when you have no idea who I am, or what I've done in the past. Sure, I could be a newcomer to programming. Then again, I could be Bjarne. How would you know? Take your righteous attitude and give it to someone else.
I agree.hurstshifter wrote:Not sure who this was being directed at but I don't think anyone in this thread has expressed the need to use every feature of the language nor has anyone claimed that they are better programmers for knowing polymorphism. As for me, I literally just learned this concept and it was interesting for me as I am very new to OO design. I think that people looking down on other programmers who over-utilize OO design techniques is just as counter-productive as the OO-abusers themselves. While it is definitely important to know when and where to use each feature of the language, it's also important to know how to use these features. I think we can agree on that.
- dandymcgee
- ES Beta Backer
- Posts: 4709
- Joined: Tue Apr 29, 2008 3:24 pm
- Current Project: https://github.com/dbechrd/RicoTech
- Favorite Gaming Platforms: NES, Sega Genesis, PS2, PC
- Programming Language of Choice: C
- Location: San Francisco
- Contact:
Re: Polymorphism
I understand inheritance perfectly, I simply said I haven't come across a reason to use it in anything I've worked on thus far.Apoc wrote: You seem to have quite a high-horse attitude. By no means did I state: 'You're not using OOP to it's fullest. Go use C'. I stated a fact, and an opinion. Then gave an example of how to use something he isn't quite familiar with. (Assuming so at least)
I also mentioned that I'm fully aware that it has it's uses and that I could have probably used it somewhere as a better alternative to whatever I did use.
I still have yet to see an example of inheritance at work as a solution to a real-world problem that doesn't involve cars or banking. Perhaps if you could think of one it would help to enlighten me as to how I could properly implement this design pattern in my own projects.

Edit: That last statement wasn't sarcasm, although it may have sounded like it. It was a genuine request for knowledge.

Falco Girgis wrote:It is imperative that I can broadcast my narcissistic commit strings to the Twitter! Tweet Tweet, bitches!
- programmerinprogress
- Chaos Rift Devotee
- Posts: 632
- Joined: Wed Oct 29, 2008 7:31 am
- Current Project: some crazy stuff, i'll tell soon :-)
- Favorite Gaming Platforms: PC
- Programming Language of Choice: C++!
- Location: The UK
- Contact:
Re: Polymorphism
We actually had an interesting post about how polymorphism and inheritance would be useful in creating your own objects in a GUI, using a standard interface for many objects, with differently implemented methods could make things a hell of a lot easier if you do it well, not to mention the reduction in the amount of code you have to write.
I'm still playing around with polymorphism, but I can't confidently say that i'm actively usingit correctly yet in my current projects.
PS: Apoc, I feel like i've heard of you before, did you used to be on the lionhead forums? I was quite active there under another alias.
I'm still playing around with polymorphism, but I can't confidently say that i'm actively usingit correctly yet in my current projects.
PS: Apoc, I feel like i've heard of you before, did you used to be on the lionhead forums? I was quite active there under another alias.
---------------------------------------------------------------------------------------
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
Re: Polymorphism
you are delusional. in no way did i implicitly state that you are a sub par programmer. just that OO MANIA, has produced a slew of sub par programmers.Apoc wrote:You seem to have quite a high-horse attitude. By no means did I state: 'You're not using OOP to it's fullest. Go use C'. I stated a fact, and an opinion. Then gave an example of how to use something he isn't quite familiar with. (Assuming so at least)avansc wrote:this is exactly why there is a influx of sub par programmers. they think that using every feature of language,
or implementing every know design pattern or design methodology makes them great programmers, or have
the attitude that if someone doesn't they are sub par programmers or should stick to a "other" language...
Using every feature of the language, or every known design pattern/methodology, usually means your code is over-complicated (especially when you consider C++).
Inheritance and polymorphism is what makes OOP so useful.
Also, I'm not sure how you have the knowledge, or ability, to call someone a sub-par programmer. Especially when you have no idea who I am, or what I've done in the past. Sure, I could be a newcomer to programming. Then again, I could be Bjarne. How would you know? Take your righteous attitude and give it to someone else.
I agree.hurstshifter wrote:Not sure who this was being directed at but I don't think anyone in this thread has expressed the need to use every feature of the language nor has anyone claimed that they are better programmers for knowing polymorphism. As for me, I literally just learned this concept and it was interesting for me as I am very new to OO design. I think that people looking down on other programmers who over-utilize OO design techniques is just as counter-productive as the OO-abusers themselves. While it is definitely important to know when and where to use each feature of the language, it's also important to know how to use these features. I think we can agree on that.
also... way to take it out of context... look at the edit. and that is specifically what i was referring to.
Some person, "I have a black belt in karate"
Dad, "Yea well I have a fan belt in street fighting"
Dad, "Yea well I have a fan belt in street fighting"
- Bakkon
- Chaos Rift Junior
- Posts: 384
- Joined: Wed May 20, 2009 2:38 pm
- Programming Language of Choice: C++
- Location: Indiana
Re: Polymorphism
My favorite use of it is the fact that all descendants can be held in a list/vector the requires an object of the original parent. It makes object management extremely simple and clean.
- programmerinprogress
- Chaos Rift Devotee
- Posts: 632
- Joined: Wed Oct 29, 2008 7:31 am
- Current Project: some crazy stuff, i'll tell soon :-)
- Favorite Gaming Platforms: PC
- Programming Language of Choice: C++!
- Location: The UK
- Contact:
Re: Polymorphism
you would store lists of pointers to the base class right?
sounds like a plan
Actually makes sense if you can just call a generic Draw() method, or something like that if the vector/list stored pointers to the different objects of the sub classes, means you could iterate through just one list as opposed to individuals, sounds a lot cleaner and more convenient.
I think you have to get the balance right though, if you're constantly focused on perfect OO design, and you're not actually getting anything out of it, you might as well be programming in a more convenient manner, but if you know how to harness the benefits of being able to treat different objects in a standard manner through a standard interface, then that's only a good thing, isn't it?
sounds like a plan

Actually makes sense if you can just call a generic Draw() method, or something like that if the vector/list stored pointers to the different objects of the sub classes, means you could iterate through just one list as opposed to individuals, sounds a lot cleaner and more convenient.
I think you have to get the balance right though, if you're constantly focused on perfect OO design, and you're not actually getting anything out of it, you might as well be programming in a more convenient manner, but if you know how to harness the benefits of being able to treat different objects in a standard manner through a standard interface, then that's only a good thing, isn't it?
---------------------------------------------------------------------------------------
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
- Bakkon
- Chaos Rift Junior
- Posts: 384
- Joined: Wed May 20, 2009 2:38 pm
- Programming Language of Choice: C++
- Location: Indiana
Re: Polymorphism
Yes, pointers. Oh god, your program would shit itself if you made copies of everything.
- dandymcgee
- ES Beta Backer
- Posts: 4709
- Joined: Tue Apr 29, 2008 3:24 pm
- Current Project: https://github.com/dbechrd/RicoTech
- Favorite Gaming Platforms: NES, Sega Genesis, PS2, PC
- Programming Language of Choice: C
- Location: San Francisco
- Contact:
Re: Polymorphism
Thanks for that. Never thought of it before (I always forget you can treat derived class objects as if they were their parent's type).Bakkon wrote:My favorite use of it is the fact that all descendants can be held in a list/vector the requires an object of the original parent. It makes object management extremely simple and clean.
Falco Girgis wrote:It is imperative that I can broadcast my narcissistic commit strings to the Twitter! Tweet Tweet, bitches!
- programmerinprogress
- Chaos Rift Devotee
- Posts: 632
- Joined: Wed Oct 29, 2008 7:31 am
- Current Project: some crazy stuff, i'll tell soon :-)
- Favorite Gaming Platforms: PC
- Programming Language of Choice: C++!
- Location: The UK
- Contact:
Re: Polymorphism
I think that's the main idea of polymorphism.
Invoking methods with the same name, but taking "tailor-made" actions, this enforces the idea of "one interface, many methods", or indeed many forms from which the term is derived from.
In theory, you should be able to treat the object as if it was the base class, but it's actions reflect that of the subclass
Invoking methods with the same name, but taking "tailor-made" actions, this enforces the idea of "one interface, many methods", or indeed many forms from which the term is derived from.
In theory, you should be able to treat the object as if it was the base class, but it's actions reflect that of the subclass
---------------------------------------------------------------------------------------
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
I think I can program pretty well, it's my compiler that needs convincing!
---------------------------------------------------------------------------------------
And now a joke to lighten to mood :D
I wander what programming language anakin skywalker used to program C3-PO's AI back on tatooine? my guess is Jawa :P
- Falco Girgis
- Elysian Shadows Team
- Posts: 10294
- Joined: Thu May 20, 2004 2:04 pm
- Current Project: Elysian Shadows
- Favorite Gaming Platforms: Dreamcast, SNES, NES
- Programming Language of Choice: C/++
- Location: Studio Vorbis, AL
- Contact:
Re: Polymorphism
That's pretty much one of the main instances that I use polymorphism.dandymcgee wrote:Thanks for that. Never thought of it before (I always forget you can treat derived class objects as if they were their parent's type).Bakkon wrote:My favorite use of it is the fact that all descendants can be held in a list/vector the requires an object of the original parent. It makes object management extremely simple and clean.
Lots of times this doesn't even do much good. If you only want to do things with certain types (and they're all in the same list) and have to ignore a massive amount, you might as well have them in separate lists to begin with.
In Elysian Shadows, everything is polymorphed back into a collidable from its original derived class. Collidables are only good for collision. The collidable vector/container has only pointers. The actual players, NPCs, enemies, items, etc are in their own respective containers, so that I can also easily loop a player against every NPC (to see if they are chatting) or every item against every player (to see if they're close enough to pick it up).
Haha, isn't that the basis of polymorphism?dandymcgee wrote:I always forget you can treat derived class objects as if they were their parent's type