Perspective
Moderator: Coders of Rage
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Perspective
OK, So I'm trying to use perspective so that things further back look smaller.
I use glFrustum and set up some locations
but when I when I move a square back it just stays the same size until it disappears.
Is there some way to enable perspective?
I use glFrustum and set up some locations
but when I when I move a square back it just stays the same size until it disappears.
Is there some way to enable perspective?
- dandymcgee
- ES Beta Backer
- Posts: 4709
- Joined: Tue Apr 29, 2008 3:24 pm
- Current Project: https://github.com/dbechrd/RicoTech
- Favorite Gaming Platforms: NES, Sega Genesis, PS2, PC
- Programming Language of Choice: C
- Location: San Francisco
- Contact:
Re: Perspective
Have you tried reading a tutorial? I know I've already linked you there once, but you should really check out http://nehe.gamedev.net/.Benjamin100 wrote:OK, So I'm trying to use perspective so that things further back look smaller.
I use glFrustum and set up some locations
but when I when I move a square back it just stays the same size until it disappears.
Is there some way to enable perspective?
Falco Girgis wrote:It is imperative that I can broadcast my narcissistic commit strings to the Twitter! Tweet Tweet, bitches!
- Ginto8
- ES Beta Backer
- Posts: 1064
- Joined: Tue Jan 06, 2009 4:12 pm
- Programming Language of Choice: C/C++, Java
Re: Perspective
glFrustrum, and perspective in general, have a tendency to be bitches. Between the two, however, I would recommend using gluPerspective over glFrustrum because it makes at least setting up the perspective less of a pain in the ass.
Also, always post relevant code if possible. We are programmers, not mind readers, so when you have a problem and say "I did x and some stuff and it doesn't work", the only clues we have are that either x or the "some stuff" caused a problem. Unfortunately, calling glFrustrum can be done multiple ways, and if you didn't call it correctly it won't work how you want it to; also, "setting up some locations" is so vague that it could mean any number of things, from simply drawing some vertices to actually doing translations, or who knows what else.
Perspective, and 3D in general, is a big beast, and you have to be careful. You have to have depth testing (unless you're going to sort all your faces before rendering), you need to make sure your perspective is set up correctly, and you may even have to deal with the pain in the ass of alpha blending. You need to have an idea of what you're doing going in, or at least you need to follow the example of someone who does. For that, I defer to dandy's suggestion of Nehe, because those tutorials are extremely useful.
Also, always post relevant code if possible. We are programmers, not mind readers, so when you have a problem and say "I did x and some stuff and it doesn't work", the only clues we have are that either x or the "some stuff" caused a problem. Unfortunately, calling glFrustrum can be done multiple ways, and if you didn't call it correctly it won't work how you want it to; also, "setting up some locations" is so vague that it could mean any number of things, from simply drawing some vertices to actually doing translations, or who knows what else.
Perspective, and 3D in general, is a big beast, and you have to be careful. You have to have depth testing (unless you're going to sort all your faces before rendering), you need to make sure your perspective is set up correctly, and you may even have to deal with the pain in the ass of alpha blending. You need to have an idea of what you're doing going in, or at least you need to follow the example of someone who does. For that, I defer to dandy's suggestion of Nehe, because those tutorials are extremely useful.
Quit procrastinating and make something awesome.
Ducky wrote:Give a man some wood, he'll be warm for the night. Put him on fire and he'll be warm for the rest of his life.
-
- Chaos Rift Newbie
- Posts: 41
- Joined: Tue Jun 21, 2011 5:39 am
- Programming Language of Choice: C++
Re: Perspective
Umm use Depth Testing?
and you have to clear that buffer too so:
If it's still the same, see if you're transformations and such are ok? S:?
Just realised that the above post already wrote this -.-
Code: Select all
glEnable(GL_DEPTH_TEST);
Code: Select all
glClear(GL_DEPTH_BUFFER_BIT);
// you can use it in one function like so:
glClear(GL_DEPTH_BUFFER_BIT | GL_COLOR_BUFFER_BIT);
Just realised that the above post already wrote this -.-
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
OK.
Here is what the function I want to render and move the blue triangle back...
gluPerspective is set at "0.0, 2.0, 0.1, 100.0"
Apparently the first parameter is supposed to be an angle. But when I set it above 0 it seems the triangle will not appear on the screen. "GL_DEPTH_TEST" is enabled, and so is the buffer. Yet it doesn't appear to move back.
Do I HAVE to use perspective AND frustum?
EDIT:Excuse the function name, it was originally a rectangle.
Here is what the function I want to render and move the blue triangle back...
Code: Select all
void rect_display(void)
{
glClearColor(0, 0, 0, 0);
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_MODELVIEW);
glPushMatrix();
glTranslatef(0, 0,translationAmount);
translationAmount+=0.0001;
glBegin(GL_TRIANGLES);
glColor3f(0.0, 0.0, 1.0); //front.
glVertex3f(0.3, 0.8, 0.2);
glVertex3f(0.3, 0.2, 0.2);
glVertex3f(0.8, 0.8, 0.2);
glEnd();
glPopMatrix();
glFlush();
glutSwapBuffers();
glutPostRedisplay();
}
Apparently the first parameter is supposed to be an angle. But when I set it above 0 it seems the triangle will not appear on the screen. "GL_DEPTH_TEST" is enabled, and so is the buffer. Yet it doesn't appear to move back.
Do I HAVE to use perspective AND frustum?
EDIT:Excuse the function name, it was originally a rectangle.
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
It seems to disappear at a certain point now, so I think it IS moving back, but it doesn't change size.
- Ginto8
- ES Beta Backer
- Posts: 1064
- Joined: Tue Jan 06, 2009 4:12 pm
- Programming Language of Choice: C/C++, Java
Re: Perspective
Post the code where you set up your projection matrix, because that seems to be the issue. You shouldn't be using both glFrustrum and gluPerspective, and it seems weird to me that calling gluPerspective with a fov angle of 0 would even work, although if you are calling both it might just be that it does nothing and yields to your glFrustrum call.
For future reference, the fov angle is the field-of-view angle, and higher values create a "fisheye lense" effect. Usually 45 is a decent setting for fov, but tweaking can yield more natural-seeming results
For future reference, the fov angle is the field-of-view angle, and higher values create a "fisheye lense" effect. Usually 45 is a decent setting for fov, but tweaking can yield more natural-seeming results
Quit procrastinating and make something awesome.
Ducky wrote:Give a man some wood, he'll be warm for the night. Put him on fire and he'll be warm for the rest of his life.
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
OK. I've been fooling around with it some more.
I added in glOrtho, which I didn't have before because I didn't know if it could work with gluPerspective. Apparently it can.
Still, I've been having problems...
I added in glOrtho, which I didn't have before because I didn't know if it could work with gluPerspective. Apparently it can.
Still, I've been having problems...
Code: Select all
void initialize_it(void) //sets up graphic stuff.
{
glClearColor(0.0, 0.0, 0.0, 0.0);
glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);
glutInitWindowSize(400,200);
glutInitWindowPosition(100,50);
glutCreateWindow("Moving Square");
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glOrtho(0.0,1.0,0.1,1.0,-1.0,1.0);
gluPerspective(0.0f,2.0f,0.1f,100.0f);
glEnable(GL_DEPTH_TEST);
glDepthFunc(GL_LEQUAL);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
Still not understanding what "load identity" does, even after looking at tutorials.
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
If it helps, I could clarify that putting the fov at almost ANYTHING above 0 seems to make it disappear.
Even 0.0001.
Thanks,
-Benjamin
Even 0.0001.
Thanks,
-Benjamin
- Ginto8
- ES Beta Backer
- Posts: 1064
- Joined: Tue Jan 06, 2009 4:12 pm
- Programming Language of Choice: C/C++, Java
Re: Perspective
Well, I see a couple things:
a) glClearColor should be after glutCreateWindow(), because until the window is created, you have no clue if there's a valid context to use.
b) glOrtho should not be there. Using orthographic and perspective projection at the same time will not behave like you think it does.
and regarding glLoadIdentity(), it sets the currently selected matrix to the "identity matrix":
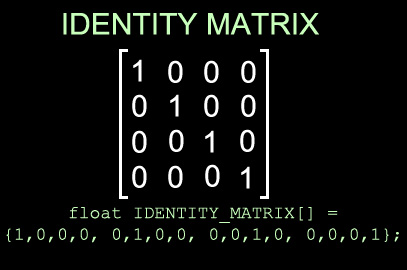
a) glClearColor should be after glutCreateWindow(), because until the window is created, you have no clue if there's a valid context to use.
b) glOrtho should not be there. Using orthographic and perspective projection at the same time will not behave like you think it does.
and regarding glLoadIdentity(), it sets the currently selected matrix to the "identity matrix":
Quit procrastinating and make something awesome.
Ducky wrote:Give a man some wood, he'll be warm for the night. Put him on fire and he'll be warm for the rest of his life.
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
OK, Thanks.
Still not moving the triangle, not sure why. That's basically it along with the main function, which initializes, displays, and then the glut main loop.
Still not moving the triangle, not sure why. That's basically it along with the main function, which initializes, displays, and then the glut main loop.
- Ginto8
- ES Beta Backer
- Posts: 1064
- Joined: Tue Jan 06, 2009 4:12 pm
- Programming Language of Choice: C/C++, Java
Re: Perspective
Well it's gotten to the point that the only way I can help you is to have the whole program. I can't see anything majorly wrong with what's been posted, as long as you fixed what I pointed out.
Quit procrastinating and make something awesome.
Ducky wrote:Give a man some wood, he'll be warm for the night. Put him on fire and he'll be warm for the rest of his life.
-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
header
cpp
Code: Select all
#include <GL/glut.h>
#include <GL/GLU.h>
#include <GL/GL.h>
void initialize_it(void)
{
glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB);
glutInitWindowSize(400,200);
glutInitWindowPosition(100,50);
glutCreateWindow("Moving Square");
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluPerspective(0.0f,2.0f,0.1f,100.0f);
glEnable(GL_DEPTH_TEST);
glDepthFunc(GL_LEQUAL);
glMatrixMode(GL_MODELVIEW);
glLoadIdentity();
}
Code: Select all
(include files)
float translationAmount=0.0;
void rect_display(void)
{
glClearColor(0, 0, 0, 0);
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT);
glMatrixMode(GL_MODELVIEW);
glPushMatrix();
glTranslatef(0, 0,translationAmount);
translationAmount+=0.0001;
glBegin(GL_TRIANGLES);
glColor3f(0.0, 0.0, 1.0);
glVertex3f(0.3, 0.8, 0.2);
glVertex3f(0.3, 0.2, 0.2);
glVertex3f(0.8, 0.8, 0.2);
glEnd();
glPopMatrix();
glFlush();
glutSwapBuffers();
glutPostRedisplay();
}
int main(int argc,char** argv)
{
glutInit(&argc,argv);
initialize_it();
glutDisplayFunc(rect_display);
glutMainLoop();
return 0;
}
- szdarkhack
- Chaos Rift Cool Newbie
- Posts: 61
- Joined: Fri May 08, 2009 2:31 am
Re: Perspective
Your frustum's near plane is 0.1 unit away from the camera. Since you do NOT change the view matrix, the camera is positioned at 0 and looking down the NEGATIVE z axis. Thus, the vertices you have defined are *behind* your view. Set a normal FoV and either flip the vertices' z coordinate to negative or translate the camera back along the positive z axis.
When i started learning OpenGL i also had a similar confusing moment. Remember 2 things:
1) By default (with the identity modelview matrix) you are looking towards the NEGATIVE half of the z axis.
2) The near and far values in gluPerspective() are *distances*, not locations. No matter how you rotate your view, these are always positive and signify how far away
from the camera you start and stop rendering objects, NOT positions on the axes.
EDIT: By the way, why is there a function implementation in your header?
When i started learning OpenGL i also had a similar confusing moment. Remember 2 things:
1) By default (with the identity modelview matrix) you are looking towards the NEGATIVE half of the z axis.
2) The near and far values in gluPerspective() are *distances*, not locations. No matter how you rotate your view, these are always positive and signify how far away
from the camera you start and stop rendering objects, NOT positions on the axes.
EDIT: By the way, why is there a function implementation in your header?

-
- ES Beta Backer
- Posts: 250
- Joined: Tue Jul 19, 2011 9:37 pm
Re: Perspective
Well, when I set the angle to something other than 0 it doesn't show anything.
This way it shows something that doesn't appear to get smaller.
This way it shows something that doesn't appear to get smaller.