Well here is my actual class i tried cutting it down in the first post for convenience with the problem :P
Code: Select all
public class e2Rect
{
private float[] vertices =
{
-1.0f, -1.0f, 0.0f,
1.0f, -1.0f, 0.0f,
1.0f, 1.0f, 0.0f,
-1.0f, 1.0f, 0.0f
};
private float[] textureCoords =
{
1.0f, 1.0f,
1.0f, 0.0f,
0.0f, 0.0f,
0.0f, 1.0f
};
public e2Vec3 Position;
public e2Vec3 Rotation;
public static int textureID;
private short[] indices = { 0, 1, 2, 0, 2, 3 };
private int vertexBuffer;
private int textureBuffer;
private int indexBuffer;
private static bool hasTexture;
public e2Rect()
{
Position = new e2Vec3();
Rotation = new e2Vec3();
int size;
GL.GenBuffers(1, out vertexBuffer);
GL.BindBuffer(BufferTarget.ArrayBuffer, vertexBuffer);
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * BlittableValueType.StrideOf(vertices)), vertices, BufferUsageHint.StaticDraw);
GL.GetBufferParameter(BufferTarget.ArrayBuffer, BufferParameterName.BufferSize, out size);
if (vertices.Length * BlittableValueType.StrideOf(vertices) != size)
throw new ApplicationException("Vertex data not uploaded correctly");
GL.GenBuffers(1, out textureBuffer);
GL.BindBuffer(BufferTarget.TextureBuffer, textureBuffer);
GL.BufferData(BufferTarget.TextureBuffer, (IntPtr)(textureCoords.Length * BlittableValueType.StrideOf(textureCoords)), textureCoords, BufferUsageHint.StaticDraw);
GL.GetBufferParameter(BufferTarget.TextureBuffer, BufferParameterName.BufferSize, out size);
if (textureCoords.Length * BlittableValueType.StrideOf(textureCoords) != size)
throw new ApplicationException("Vertex data not uploaded correctly");
GL.GenBuffers(1, out indexBuffer);
GL.BindBuffer(BufferTarget.ElementArrayBuffer, indexBuffer);
GL.BufferData(BufferTarget.ElementArrayBuffer, (IntPtr)(indices.Length * sizeof(short)), indices, BufferUsageHint.StaticDraw);
GL.GetBufferParameter(BufferTarget.ElementArrayBuffer, BufferParameterName.BufferSize, out size);
if (indices.Length * sizeof(short) != size)
throw new ApplicationException("Element data not uploaded correctly");
}
public void Draw()
{
GL.PushMatrix();
GL.Color3(System.Drawing.Color.White);
GL.FrontFace(FrontFaceDirection.Ccw);
GL.Enable(EnableCap.CullFace);
GL.CullFace(CullFaceMode.Back);
GL.Enable(EnableCap.AlphaTest);
GL.AlphaFunc(AlphaFunction.Greater, 0.1f);
GL.Translate(Position.X, Position.Y, Position.Z);
GL.Rotate(Rotation.X, 1f, 0.0f, 0.0f);
GL.Rotate(Rotation.Y, 0.0f, 1f, 0.0f);
GL.Rotate(Rotation.Z, 0.0f, 0.0f, 1f);
//GL.BindBuffer(BufferTarget.ArrayBuffer, vertexBuffer);
GL.VertexPointer(3, VertexPointerType.Float, 3 * sizeof(float), IntPtr.Zero);
GL.EnableClientState(ArrayCap.VertexArray);
GL.Enable(EnableCap.Blend);
GL.BlendFunc(BlendingFactorSrc.One, BlendingFactorDest.OneMinusSrcAlpha);
if (hasTexture)
{
GL.Enable(EnableCap.Texture2D);
GL.BindTexture(TextureTarget.Texture2D, textureID);
//GL.BindBuffer(BufferTarget.TextureBuffer, textureBuffer);
//GL.TexCoordPointer(2, TexCoordPointerType.Float, 2 * sizeof(float), new IntPtr(0));
GL.TexCoordPointer(2, TexCoordPointerType.Float, 4 * sizeof(float), new IntPtr(8));
GL.EnableClientState(ArrayCap.TextureCoordArray);
}
GL.BindBuffer(BufferTarget.ElementArrayBuffer, indexBuffer);
GL.DrawElements(BeginMode.Triangles, indices.Length, DrawElementsType.UnsignedShort, IntPtr.Zero);
if (hasTexture)
GL.DisableClientState(ArrayCap.TextureCoordArray);
GL.DisableClientState(ArrayCap.VertexArray);
GL.Disable(EnableCap.Texture2D);
GL.Disable(EnableCap.CullFace);
GL.Disable(EnableCap.Blend);
GL.PopMatrix();
}
public static void loadTexture()
{
textureID = e2Loader.LoadImage(e2Textures.Odin3);
hasTexture = true;
}
}
Now this is from my knowledge of drawing in OpenTK like this so far, So i don't know if it is correct or not.
Code: Select all
//Generate a buffer...
GL.GenBuffers(1, out vertexBuffer);
//Bind said buffer...
GL.BindBuffer(BufferTarget.ArrayBuffer, vertexBuffer);
//Write data to the currently bound buffer,
//1st param is the buffer type.
//Same as a byte array, the second parameter is the length in bytes of the buffer data
//so i have 12 vertices all floats, float in bytes is 4 so 48 bytes in total, or vertices.Length * sizeof(float);
//last param is telling GL how to draw it, not that GL will always listen...
GL.BufferData(BufferTarget.ArrayBuffer, (IntPtr)(vertices.Length * BlittableValueType.StrideOf(vertices)), vertices, BufferUsageHint.StaticDraw);
//draw
//now point to the ArrayBuffer
//1st parameter is the size to read
//2nd is the type float in this case
//3rd is the stride in bytes so its reading 3 floats so 12 bytes
//4th is the Pointer in bytes where to start reading
GL.VertexPointer(3, VertexPointerType.Float, 3 * sizeof(float), IntPtr.Zero);
GL.EnableClientState(ArrayCap.VertexArray);
Now the thing is i wrote the data to the Buffers so it has the references to each array
now if its acting as my whole class is being read then
GL.TexCoordPointer(2, TexCoordPointerType.Float, 2 * sizeof(float), new IntPtr(48));
would work since both arrays are at the top and the textureCoords is 48 bytes (vertices) from the top
but soon found out after my last post that, that can't be right since my class is nullable and GL would throw an error since it only takes non-nullable parameters.
so in fact
GL.TexCoordPointer(2, TexCoordPointerType.Float, 2 * sizeof(float), new IntPtr(0));
should be the correct way since it was referenced to bind to the TextureBuffer on init of the class
but it's acting like the textureCoords are bound to the vertexBuffer and only reading those
GL.BindBuffer(BufferTarget.ArrayBuffer, vertexBuffer);
GL.TexCoordPointer(2, TexCoordPointerType.Float, 2 * sizeof(float), new IntPtr(0));
and i found this out by debugging ofcourse...
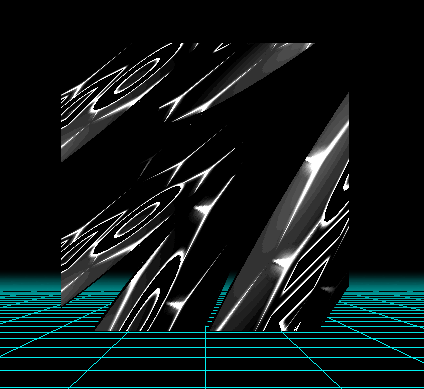
- OdinScreeny1.png (39.28 KiB) Viewed 4145 times
which is
GL.TexCoordPointer(2, TexCoordPointerType.Float, 2 * sizeof(float), new IntPtr(0));
and
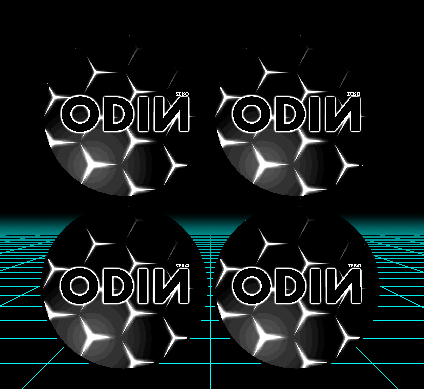
- OdinScreeny2.png (40.84 KiB) Viewed 4145 times
is
GL.TexCoordPointer(3, TexCoordPointerType.Float, 3 * sizeof(float), new IntPtr(0));
now i don't know how it get's to the vertex buffer when the texture pointer is to the texture buffer lol
i hope i explained that well enough :]