OpenGL Failure
Posted: Mon Aug 20, 2012 9:08 am
Here's the FULL source code:
And this it what this code produces:
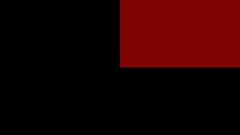
I have no idea why it does this. Just FYI I believe I recently installed an updated video card driver so that may be the cause but IDK. Please help!
Code: Select all
#include "SDL.h"
#include "SDL_opengl.h"
#pragma comment( lib, "SDL.lib" )
#pragma comment( lib, "SDLmain.lib" )
#pragma comment( lib, "OpenGL32.lib" )
int main( int num, char **blarg )
{
SDL_Init( SDL_INIT_EVERYTHING );
SDL_GL_SetAttribute( SDL_GL_RED_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_GREEN_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_BLUE_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_ALPHA_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_BUFFER_SIZE, 32 );
SDL_GL_SetAttribute( SDL_GL_DEPTH_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_ACCUM_RED_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_ACCUM_GREEN_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_ACCUM_BLUE_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_ACCUM_ALPHA_SIZE, 8 );
SDL_GL_SetAttribute( SDL_GL_DOUBLEBUFFER, 1 );
glClearColor( 0.0F, 0.0F, 0.0F, 0 );
glClearDepth( 1.0f );
glViewport( 0, 0, 1280, 720 );
glMatrixMode( GL_PROJECTION );
glLoadIdentity();
glMatrixMode( GL_MODELVIEW );
glLoadIdentity();
SDL_SetVideoMode( 1280, 720, 32, SDL_OPENGL );
glOrtho( 0, 1280, 720, 0, 1, -1 );
glEnable( GL_BLEND );
glBlendFunc( GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA );
while( 1 )
{
glClear( GL_COLOR_BUFFER_BIT );
glLoadIdentity();
glColor4ub( 255, 8, 8, 128 );
glBegin( GL_QUADS );
glVertex2f( 0.0F, 0.0F );
glVertex2f( 1.0F, 0.0F );
glVertex2f( 1.0F, 1.0F );
glVertex2f( 0.0F, 1.0F );
glEnd();
SDL_GL_SwapBuffers();
}
SDL_Quit();
return 0;
}
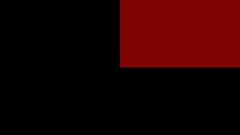
I have no idea why it does this. Just FYI I believe I recently installed an updated video card driver so that may be the cause but IDK. Please help!